Capturing logs from the device-under-test#
You can configure the Stb-tester Node to capture logs from the device-under-test during test-runs and manual control recordings.
This is configured using a pytest fixture that allows you to run code before
and after a test-run to start and stop log capture. Stb-tester will look in your
test-pack at tests/conftest.py
for a fixture called capture_dut_logs
.
How you implement capture_dut_logs
depends on the type of device you are
testing, and other details of your system (for example, you might also want to
capture server logs).
If your test-farm has many different types of devices, you can use
stbt.get_config("device_under_test", "device_type")
to check the device
type, and start the appropriate log capture process. To configure the
device_type
of each Stb-tester Node in your test-farm, see
Node-specific configuration files.
For example:
import pytest
import stbt
@pytest.fixture(autouse=True)
def capture_dut_logs():
device_type = stbt.get_config("device_under_test", "device_type", None)
match device_type:
case "firetv":
# start adb capture here
yield
# stop adb capture here
case "roku":
# start roku capture here
yield
# stop roku capture here
case _:
print(f"No log capture for device type {device_type}.")
yield
Note that conftest.py
is a special file that pytest imports automatically.
autouse=True
means that every test will automatically use the fixture; you
don’t have to explicitly request it in each test. For more information see
pytest’s documentation on fixtures and conftest.py.
Android log capture#
For Android devices you can use Stb-tester’s stbt.android.AdbDevice.logcat
Python API to capture logs using adb logcat
.
To save logs to a file called “logcat.log”, add this fixture to
tests/conftest.py
:
import pytest
from stbt.android import AdbDevice
@pytest.fixture(autouse=True)
def capture_dut_logs():
adb = AdbDevice()
with adb.logcat("logcat.log"):
yield
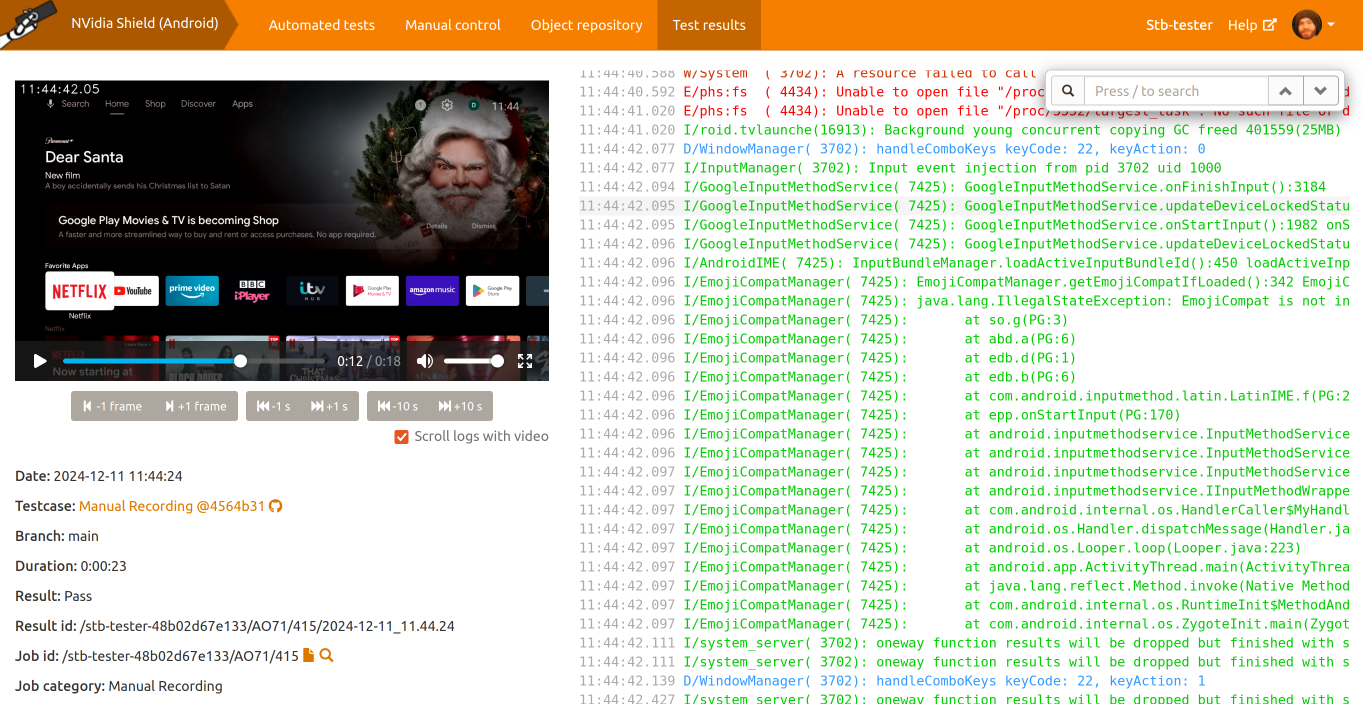
To save logcat logs inline into the Stb-tester log (so you can view it in Stb-tester’s Interactive log viewer with timestamps synchronised to the video recording) use this fixture:
import pytest
from stbt.android import AdbDevice
@pytest.fixture(autouse=True)
def capture_dut_logs():
# Print logcat logs to standard output; Stb-tester automatically timestamps
# each line and saves it to "stbt.log". logcat's "brief" format disables
# logcat's own timestamping, to avoid double timestamps.
adb = AdbDevice()
with adb.logcat(
"/dev/stdout",
["-v", "brief", "-v", "color", "-v", "printable"]):
yield
Roku log capture#
Roku devices expose a debug console over the network. You can capture logs
from this console using Stb-tester’s stbt.Roku.save_logs
Python API. For
example, add this fixture to tests/conftest.py
:
import pytest
import stbt
@pytest.fixture(autouse=True)
def capture_dut_logs():
roku = stbt.Roku.from_config()
with roku.save_logs("roku.log"):
yield