Regions and Masks#
Regions#
Some stbt APIs take an optional region parameter that allows you to
restrict the search to a rectangular region of the video frame. For example, if
you’re using stbt.match
to find the focused menu element in the YouTube app
shown below, you can restrict the search to the left part of the screen where
the menu is:
stbt.match("focus.png", region=stbt.Region(x=0, y=0, width=330, height=720))
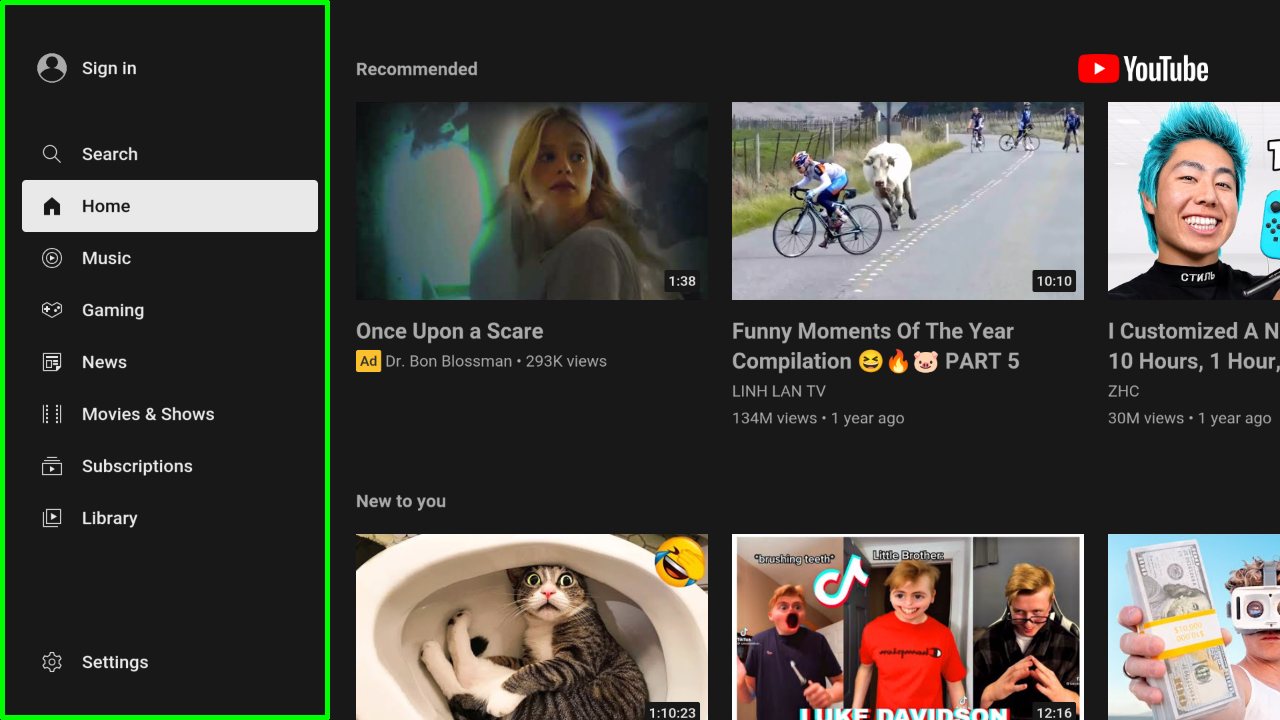
YouTube menu, with the region highlighted in green#
The coordinates start from the top left of the image, so x=0, y=0
is the
top left corner and x=1280, y=720
is the bottom right corner of the frame.
Create a Region
by specifying x, y, width, height
or x, y, right,
bottom
. Stb-tester’s image processing is done at 720p, regardless of the
output resolution of your device-under-test; Stb-tester rescales the captured
video to 720p, so you don’t need to change the coordinates in your Python code
to test different output resolutions.
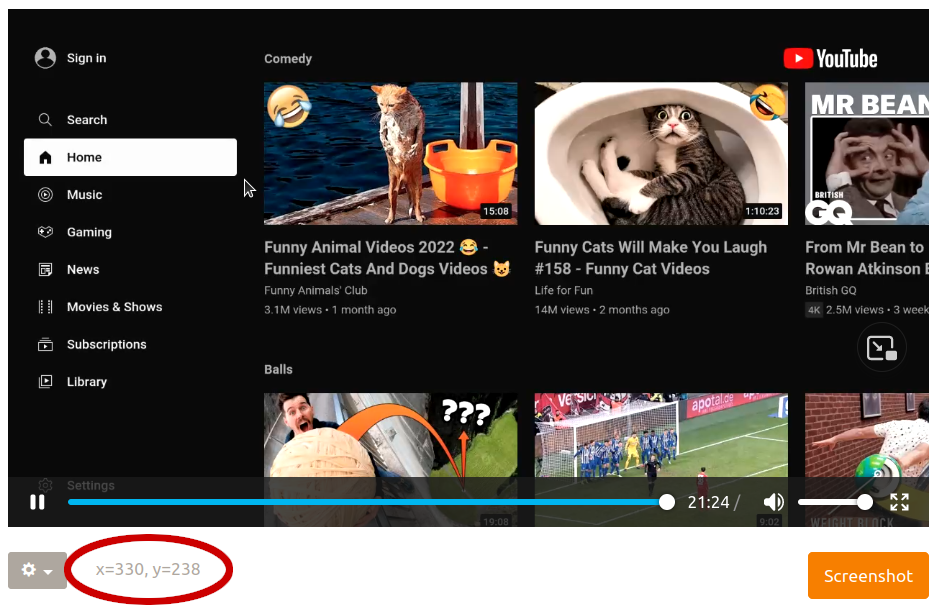
In the Stb-tester Portal, hover your cursor over the live video to see the coordinates to use in your Region.#
stbt.Region.ALL
means the entire frame; it’s the default value for the
region
parameter of these APIs.
Masks#
Some stbt APIs take a parameter called mask instead of region. The mask
parameter accepts a Region
(just like the region
parameter) but it also
accepts more complex masks.
Conceptually, a mask is a black and white image where white pixels specify which parts of the frame to check, and black pixels specify which parts of the frame to ignore.
For example, we can use a mask with stbt.is_screen_black
to detect a channel
change. We need to mask out certain GUI elements that appear even when the
content is black:
frame |
mask |
is_screen_black(frame, mask) |
---|---|---|
![]() |
None |
False |
![]() |
![]() |
True |
You can create a mask in two different ways:
Create a black-and-white PNG image in an image editor, commit the image to git, and give the image’s filename to the
mask
parameter, for examplemask="channel-change-mask.png"
. The image must be the same size as the frame, typically 1280x720.
Or,
Construct the mask in Python code by adding or subtracting Regions. For example, the mask shown above can be created with the following code:
channel_patch = stbt.Region(x=570, y=264, right=715, bottom=450) info_bar = stbt.Region(x=0, y=520, right=1280, bottom=690) mask = stbt.Region.ALL - channel_patch - info_bar
Adding 2 or more Regions together creates a mask with the pixels inside those Regions selected; all other pixels ignored.
Subtracting a Region removes that Region’s pixels from the mask (so those pixels will be ignored).
You can invert (mask out) a Region using the ~
operator. For example, the
following masks are equivalent:
mask1 = ~channel_patch
mask2 = stbt.Region.ALL - channel_patch